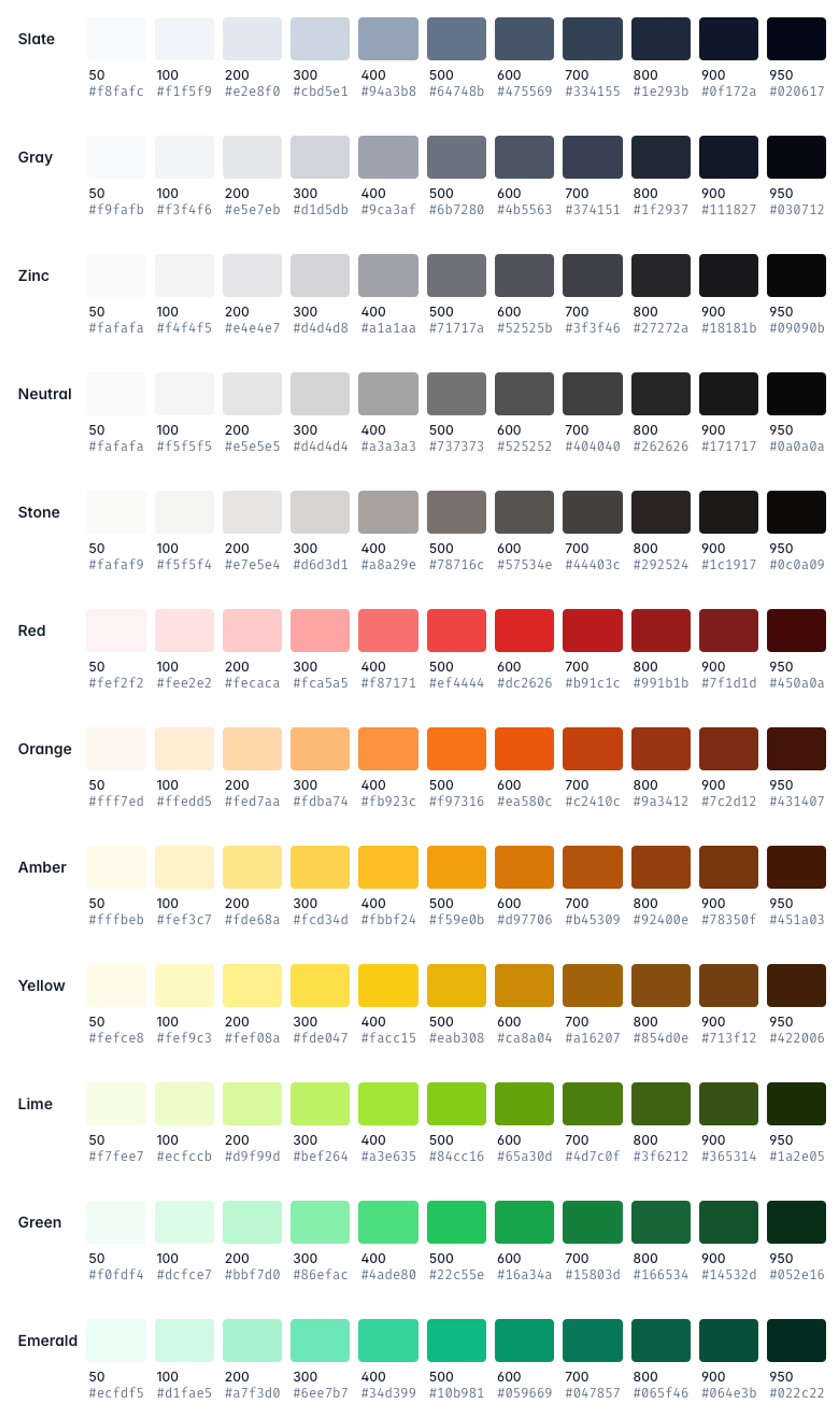
You're knee-deep in a web project, leveraging the power of TailwindCSS for rapid development. But as you scroll through the seemingly endless color options, you can't shake the feeling that you're drowning in a sea of unused styles. Sound familiar? You're not alone. Many developers struggle with TailwindCSS's vast color palette, leading to bloated CSS files and decreased performance.
But what if you could harness all the flexibility of TailwindCSS while maintaining a lean, efficient codebase?
The solution lies in mastering your tailwind.config.js
file. By learning to customize and optimize your color configurations, you can create a streamlined, project-specific color palette that enhances both your design consistency and your app's performance.
In this guide, I'll walk you through practical steps to tame the TailwindCSS color beast. Whether you're a TailwindCSS novice or a seasoned pro, you'll discover valuable techniques to refine your workflow and boost your productivity. Ready to take control of your color palette? Let's dive in!
Understanding TailwindCSS Color System
Have you ever wondered why TailwindCSS comes with so many colors out of the box? While this vast palette offers incredible flexibility, it can also lead to unexpected challenges. Let's take a closer look.
TailwindCSS provides a default color palette that includes a wide range of colors and shades. From blues to reds, and everything in between, you've got options galore.
But here's the million-dollar question: do you really need all these colors for your project?
Consider this: with such a vast array of colors at your fingertips, it's easy to fall into the trap of inconsistency. Do you find yourself debating between slate or stone for neutral tones? Violet or purple for your accents? This abundance of choice, while offering flexibility, can lead to design inconsistencies if not managed carefully.
In web design, color consistency is key to creating a cohesive user experience. It helps establish your brand identity, guides users through your interface, and creates a sense of harmony across your site. But when you're juggling too many color options, maintaining this consistency becomes challenging.
Moreover, a sprawling color palette can complicate decision-making during the design process. Designers and developers might spend unnecessary time debating subtle color differences instead of focusing on more critical aspects of the user experience.
So, the question becomes: how do we harness the power of TailwindCSS's color system while maintaining a focused, consistent design language? The answer lies in thoughtful configuration and curation of your color palette.
Optimizing Your Tailwind Config
In this section, we'll explore various techniques to customize and optimize your TailwindCSS configuration (tailwind.config.js
) for a leaner, more efficient color system.
Disable Default Colors
Think of it like this: having too many colors in your palette is like being a chef with an overstocked spice rack.
Sure, you have every flavor at your disposal, but using them all doesn't necessarily make for a better dish.
In fact, it might lead to a confusing and inconsistent flavor profile.
The best approach is to override the default color palette and just include the colors you want.
tailwind.config.js
const colors = require('tailwindcss/colors');
/** @type {import('tailwindcss').Config} */
module.exports = {
theme: {
colors: {
transparent: 'transparent',
current: 'currentColor',
white: colors.white,
black: colors.black,
blue: colors.blue,
red: colors.red,
violet: colors.violet,
gray: colors.stone, // Set stone as gray color
}
}
}
Pass Custom Colors
So, how do we solve this color conundrum? The answer lies in your tailwind.config.js file. This powerful configuration file is your ticket to a leaner, meaner TailwindCSS setup.
Let's start with the basics. In your tailwind.config.js, you can specify exactly which colors you want to use and disable any of the default colors.
tailwind.config.js
/** @type {import('tailwindcss').Config} */
module.exports = {
theme: {
colors: {
blue: '#1fb6ff',
purple: '#7e5bef',
orange: '#ff9800',
}
}
}
Keep in mind if you pass a hex value to the color property, you'll lose the ability to pass color opacity.
Leveraging Tailwind's Color Functions
But wait, there's more! What if you want a consistent color scheme with various shades?
Enter Tailwind's color palette functions.
These nifty tools allow you to generate a full range of shades from a base color.
tailwind.config.js
const colors = require('tailwindcss/colors')
/** @type {import('tailwindcss').Config} */
module.exports = {
theme: {
extend: {
colors: {
primary: colors.blue,
secondary: colors.gray,
}
}
}
}
With this setup, you now have access to a full range of blue and gray shades, all consistently named (e.g., primary-100
, primary-200
, etc.). It's like having a color wizard at your fingertips!
Remember to include all the file paths where you use Tailwind classes. It's a small step that can lead to big performance gains.
Semantic Color Naming
Let's shift gears and talk about naming convention.
Have you ever looked at your code and wondered what text-gray-700
actually represents in your design system?
This is where semantic color naming comes to the rescue.
Instead of using generic color names, why not use names that actually describe the color's purpose?
tailwind.config.js
/** @type {import('tailwindcss').Config} */
module.exports = {
theme: {
extend: {
colors: {
brand: '#1fb6ff',
accent: '#7e5bef',
warning: '#ff9800',
}
}
}
}
Now, text-brand
or bg-warning
instantly communicates the purpose of the color in your design.
It's like turning your color names into a form of documentation!
Advanced Configuration Techniques
CSS Variables
For the power users out there, let's explore some advanced techniques. Have you considered using CSS variables for dynamic theming? Or perhaps extending default color palettes for more granular control?
Before you could start using CSS variables on your Tailwind config, you'll need to define these variables on your main CSS file.
Note: Define your CSS variables as channels with no color space function
main.css
@tailwind base;
@tailwind components;
@tailwind utilities;
@layer base {
:root {
--color-primary: 255 115 179;
--color-secondary: 111 114 185;
/* ... */
}
}
Don't forget to pass alpha-value
on your colors palette.
tailwind.config.js
module.exports = {
theme: {
colors: {
primary: 'rgb(var(--color-primary) / <alpha-value>)',
secondary: 'rgb(var(--color-secondary) / <alpha-value>)',
}
}
}
When defining colors this way, you'll be able to pass opacity directly to the color clases such as text-primary/50
, text-secondary/10
.
Set Neutral Color
Tailwind has lots of neutral colors to choose from zinc
, slate
, stone
, etc. I tend to forgot which color I use for my project and ended up with a mixed of inconsistent colors.
Instead of that, you could pass the neutral color as gray
and use it throughout your project.
tailwind.config.js
const colors = require('tailwindcss/colors');
/** @type {import('tailwindcss').Config} */
module.exports = {
theme: {
colors: {
transparent: 'transparent',
current: 'currentColor',
white: colors.white,
black: colors.black,
blue: colors.blue,
red: colors.red,
violet: colors.violet,
gray: colors.stone, // Set stone as gray color
}
}
}
In the example above, we're passing colors.stone
as gray
.
With this, there's no guessing which neutral color you're using on your project and your editor will be smart enough not to display those classes.
Before
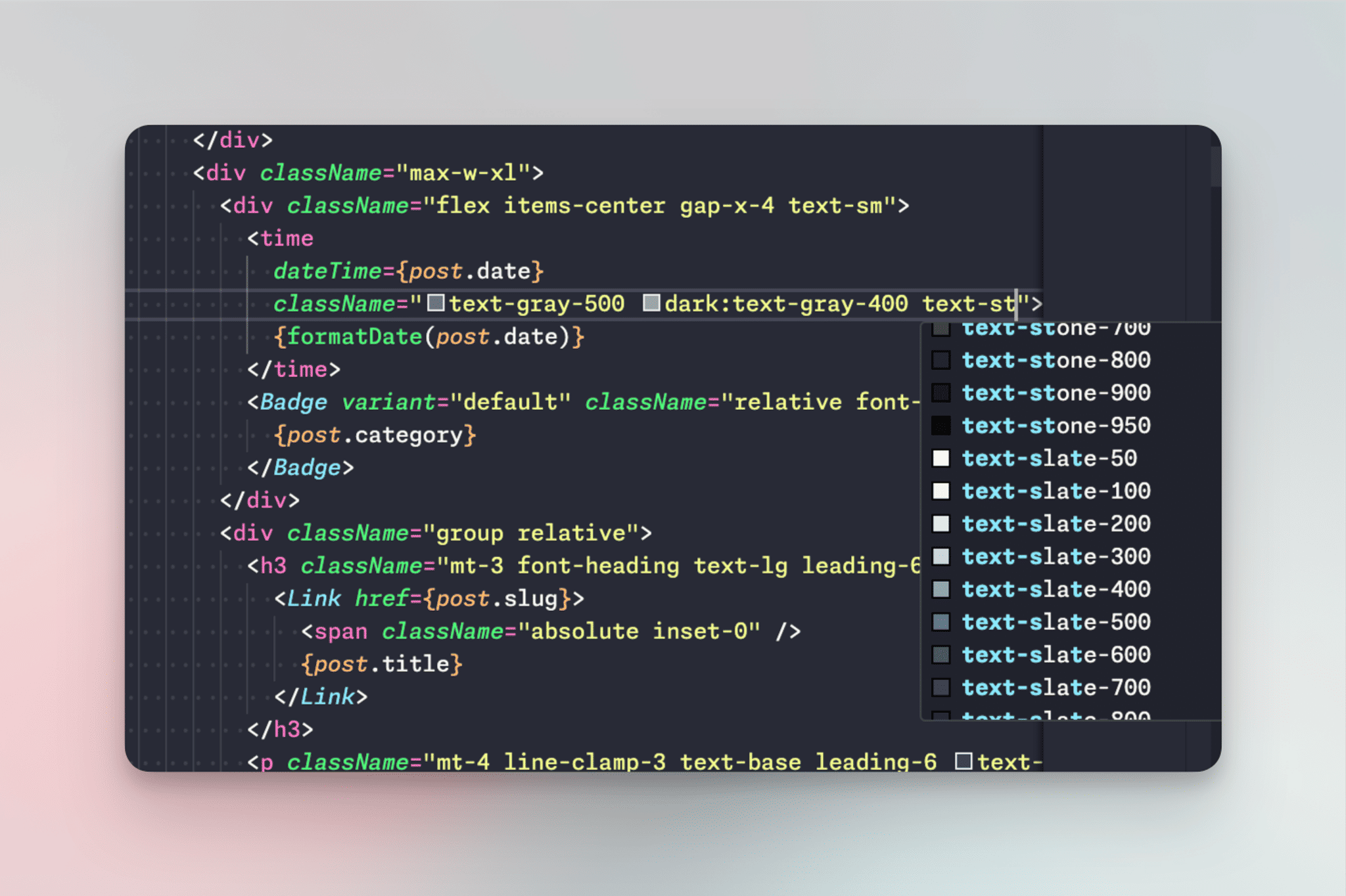
After
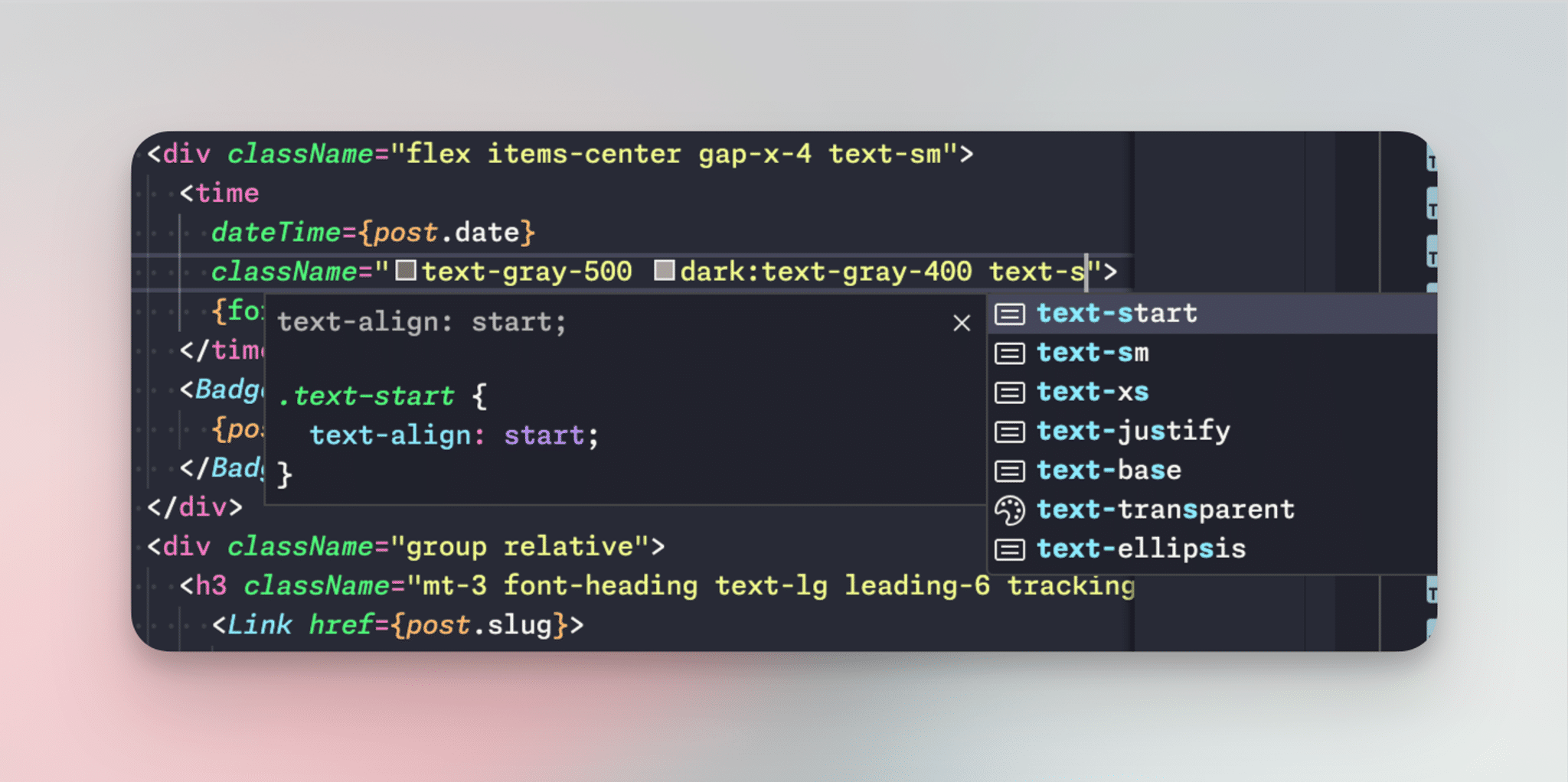
Conclusion
As we've explored in this guide, mastering TailwindCSS color configuration is a game-changer for your web development workflow. By implementing these optimization techniques, you're not just reducing file sizes – you're creating a more maintainable, performant, and visually consistent project.
Remember, the key to success lies in thoughtful customization. Take the time to analyze your project's needs, create a focused color palette, and leverage Tailwind's powerful configuration options. Your future self (and your users) will thank you for the leaner codebase and faster load times.
So, what's your next step? We encourage you to open up your tailwind.config.js file and start experimenting with these techniques. Streamline your color options, implement semantic naming, and watch as your development process becomes smoother and more efficient. Happy coding, and here's to building beautiful, performant web applications with TailwindCSS!